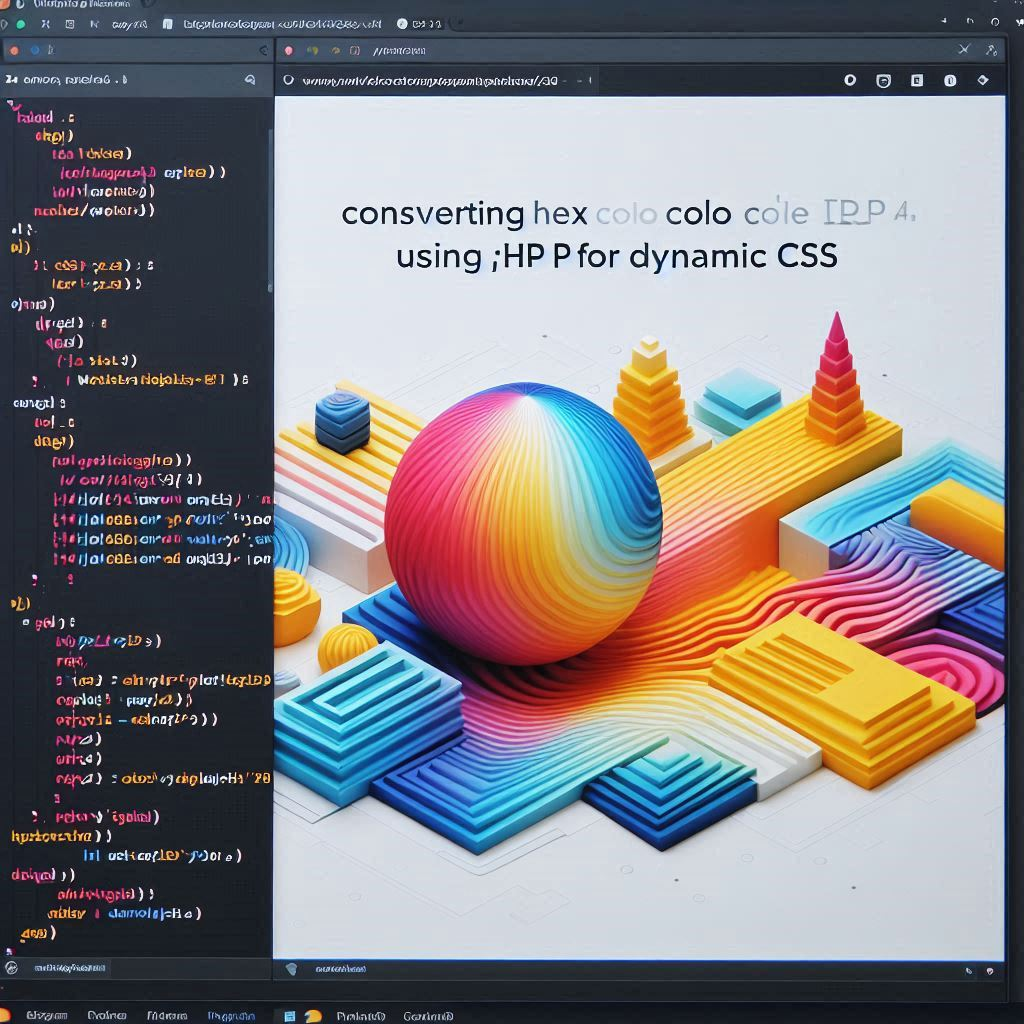
Dynamic CSS: Converting Hex to RGBA using PHP
Introduction
Managing colors dynamically in web development can significantly improve efficiency and ensure design consistency. This is particularly important for themes, such as WordPress themes, where design elements may need to adapt based on user interaction, branding changes, or theme modifications. One common requirement is to convert hex color codes to RGBA to allow for different opacity levels. In this tutorial, we’ll demonstrate how to achieve this using PHP to convert hex color codes to RGB and then create RGBA color values for use in CSS.
At agustealo.com, I faced the challenge of managing a large palette of colors dynamically across different elements of theming, especially when needing various opacity levels of the same base colors. This approach not only simplifies our CSS but also keeps it DRY (Don’t Repeat Yourself), maintainable, and adaptable.
Table of Contents
- Introduction
- Prerequisites
- Setup
- Creating the PHP Function
- Using the Function in CSS
- Example Use Case
- Advanced Techniques
- Conclusion
Prerequisites
Before we dive into the solution, ensure you have the following:
- Basic knowledge of PHP and CSS.
- A working WordPress theme where you can add custom PHP and CSS.
Setup
Step 1: Prepare Your WordPress Theme
Ensure you have the following files in your WordPress theme:
functions.php
: For adding custom PHP functions.style.css
: For basic CSS styles.theme.css
: For theme-specific CSS styles.widgets.css
: For widget and sidebar-specific CSS styles.
Creating the PHP Function
First, we’ll create a PHP function to convert hex color codes to RGB. This function will be used to generate RGBA colors dynamically in our CSS.
<?php
function hex2rgb($hex) {
$hex = str_replace("#", "", $hex);
if(strlen($hex) == 3) {
$r = hexdec(substr($hex,0,1).substr($hex,0,1));
$g = hexdec(substr($hex,1,1).substr($hex,1,1));
$b = hexdec(substr($hex,2,1).substr($hex,2,1));
} else {
$r = hexdec(substr($hex,0,2));
$g = hexdec(substr($hex,2,2));
$b = hexdec(substr($hex,4,2));
}
return implode(", ", [$r, $g, $b]);
}
?>
This function accepts a hex color code as input and returns a string containing the RGB values.
Using the Function in CSS
To use this function in CSS, follow these steps:
Step 1: Define Your PHP Variables
In your functions.php
file, define the color variables you want to use:
<?php
$primary_color = "#3498db";
$secondary_color = "#2ecc71";
$border_color = "#e74c3c";
?>
Step 2: Generate CSS Variables
Use the hex2rgb
function to convert these hex colors to RGB and define the CSS variables:
<style>
:root {
--primary-color: <?php echo $primary_color; ?>;
--secondary-color: <?php echo $secondary_color; ?>;
--border-color: <?php echo $border_color; ?>;
/* RGB Colors */
--primary-color-rgb: <?php echo hex2rgb($primary_color); ?>;
--secondary-color-rgb: <?php echo hex2rgb($secondary_color); ?>;
--border-color-rgb: <?php echo hex2rgb($border_color); ?>;
/* Transparent Versions */
--primary-color-transparent: rgba(var(--primary-color-rgb), 0.1); /* 10% opacity */
--secondary-color-transparent: rgba(var(--secondary-color-rgb), 0.1); /* 10% opacity */
--border-color-transparent: rgba(var(--border-color-rgb), 0.1); /* 10% opacity */
}
</style>
Step 3: Use CSS Variables in Your Styles
In your CSS files (style.css
, theme.css
, and widgets.css
), use these variables to style your elements:
body {
font-family: var(--primary-font);
color: var(--primary-color);
}
.search-form {
background-color: var(--border-color-transparent);
}
.button {
background-color: var(--primary-color);
color: var(--secondary-color);
}
.button:hover {
background-color: var(--primary-color-transparent);
}
Example Use Case
HTML
Add the following HTML to your WordPress theme file:
<form class="search-form" action="/" method="get">
<input class="search-field" type="text" name="s" placeholder="Search...">
<button class="wp-block-search__button" type="submit">Search</button>
</form>
<button class="button">Click Me</button>
CSS
Use the CSS defined earlier to style the form and button:
.search-form {
display: flex;
padding: 8px;
border: 2px solid var(--border-color);
border-radius: 4px;
background-color: var(--border-color-transparent);
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.1);
transition: box-shadow 0.3s ease-in-out;
}
.search-field {
flex-grow: 1;
margin-right: 8px;
padding: 10px;
border: none;
border-radius: 4px 0 0 4px;
font-size: 1rem;
outline: none;
background-color: #fff;
color: #333;
transition: background-color 0.3s ease-in-out, color 0.3s ease-in-out;
}
.search-field::placeholder {
color: #999;
}
.search-field:focus {
background-color: #fff;
color: #333;
box-shadow: 0 0 0 2px var(--link-color);
}
.wp-block-search__inside-wrapper .wp-block-search__button {
padding: 10px 20px;
border: none;
border-radius: 0 4px 4px 0;
background-color: var(--button-bg-color);
color: var(--button-text-color);
cursor: pointer;
transition: background-color 0.3s ease-in-out, color 0.3s ease-in-out;
}
.wp-block-search__inside-wrapper .wp-block-search__button:hover {
background-color: var(--button-hover-color);
color: #fff;
}
PHP
Ensure the PHP to generate the styles is included in your theme’s header.php or a similar file:
<?php
$primary_color = "#3498db";
$secondary_color = "#2ecc71";
$border_color = "#e74c3c";
?>
<style>
:root {
--primary-color: <?php echo $primary_color; ?>;
--secondary-color: <?php echo $secondary_color; ?>;
--border-color: <?php echo $border_color; ?>;
/* RGB Colors */
--primary-color-rgb: <?php echo hex2rgb($primary_color); ?>;
--secondary-color-rgb: <?php echo hex2rgb($secondary_color); ?>;
--border-color-rgb: <?php echo hex2rgb($border_color); ?>;
/* Transparent Versions */
--primary-color-transparent: rgba(var(--primary-color-rgb), 0.1); /* 10% opacity */
--secondary-color-transparent: rgba(var(--secondary-color-rgb), 0.1); /* 10% opacity */
--border-color-transparent: rgba(var(--border-color-rgb), 0.1); /* 10% opacity */
}
</style>
Advanced Techniques
Handling Multiple Opacity Levels
You can extend the function to handle multiple opacity levels by creating additional CSS variables:
<style>
:root {
--primary-color-transparent-10: rgba(var(--primary-color-rgb), 0.1); /* 10% opacity */
--primary-color-transparent-20: rgba(var(--primary-color-rgb), 0.2); /* 20% opacity */
--primary-color-transparent-30: rgba(var(--primary-color-rgb), 0.3); /* 30% opacity */
/* Add more as needed */
}
</style>
Using SCSS or LESS
If you are using a CSS preprocessor like SCSS or LESS, you can create functions to handle the conversion and use them within your stylesheets.
Example SCSS Function
@function hex2rgba($color, $alpha) {
@if (str-length($color) == 4) {
$r: unquote("0x#{str-slice($color, 2, 2)}");
$g: unquote("0x#{str-slice($color, 3, 3)}");
$b: unquote("0x#{str-slice($color, 4, 4)}");
} @else {
$r: unquote("0x#{str-slice($color, 2, 3)}");
$g
: unquote("0x#{str-slice($color, 4, 5)}");
$b: unquote("0x#{str-slice($color, 6, 7)}");
}
@return rgba($r, $g, $b, $alpha);
}
Using the SCSS Function
body {
background-color: hex2rgba(#3498db, 0.1);
}
Conclusion
By using PHP to convert hex colors to RGB and creating dynamic CSS variables, you can efficiently manage and use colors in your WordPress theme. This approach helps maintain consistency, simplifies color management, and allows for easy adjustments to transparency and other properties.
Feel free to extend this approach with more colors and variables to suit your theme’s needs. This method ensures your CSS remains DRY (Don’t Repeat Yourself) and maintainable.
By implementing these techniques on agustealo.com, we’ve streamlined our theming process, ensuring a more consistent and dynamic user experience. This approach can be particularly beneficial for developers looking to enhance their theme’s flexibility and maintainability.