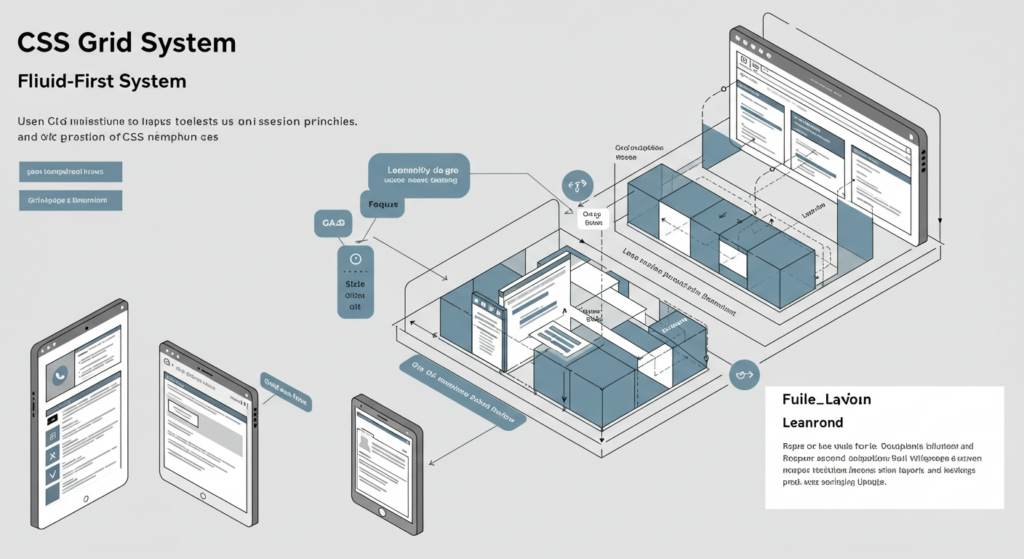
1. Introduction
This CSS Grid System is designed to be lightweight, scalable, and modular, following modern web development best practices. It uses:
- CSS Variables for easy customization
- A mobile-first approach to enhance performance and UX
- Utility classes for spacing, layout, and alignment
- Flexbox and Grid support to provide fluid and adaptive layouts
- Minimal CSS footprint, making it ideal for fast-loading web pages
This guide will help you understand, implement, and extend the system efficiently.
- Global Reset & Variables
- Container System
- Rows & Columns
- Responsive Breakpoints
- Spacing Utilities (Margins & Padding)
- Layout & Flow Utilities (Flex & Grid Helpers)
- Responsive Order & Flex Controls
Below youâll find a detailed explanation of each section along with use cases.
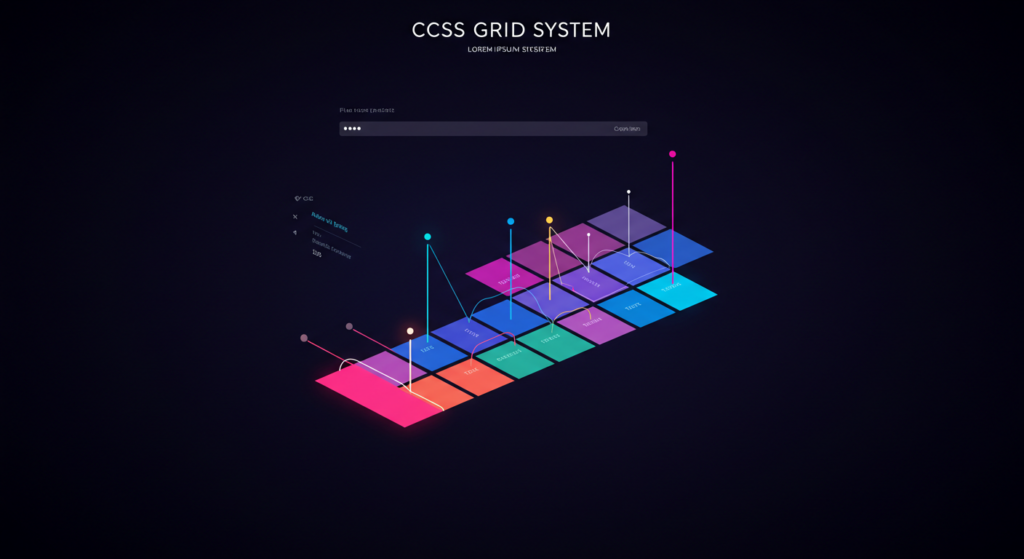
1. Global Reset & Variables
Global Reset
html {
box-sizing: border-box;
}
*, *::before, *::after {
box-sizing: inherit;
margin: 0;
padding: 0;
}
Purpose:
This ensures every element, its pseudo-elements, and inherited elements use the same box model. It eliminates default browser margins and paddings, providing a clean slate to work from.
Use Case:
Always include a reset to prevent unexpected spacing and sizing issues. This is essential for a consistent design across different browsers.
Global Variables
:root {
--container-width: 100%;
--container-max-width: 1200px; /* Default max width */
--container-fluid-max-width: 100%;
--grid-gap: 20px; /* Default gap between columns */
--grid-columns: 12; /* Defines a 12-column layout */
--base-padding: 1rem;
}
Purpose:
CSS variables are declared in the :root
so theyâre globally available. They make it easy to adjust key values (like container widths, grid gaps, and the number of columns) in one place.
Use Case:
When you need to change the overall layout (for example, increasing the gap or setting a new max-width), adjust these variables instead of hunting through multiple rules.
2. Container System
Container Classes
.container,
.container-fluid,
.container-narrow,
.container-wide {
width: var(--container-width);
margin: 0 auto;
padding-left: var(--grid-gap);
padding-right: var(--grid-gap);
}
Purpose:
Containers center your content and provide horizontal padding (gutters). Different container classes let you choose between fixed-width, fluid, narrow, or wide content areas.
.container
: Has a maximum width (default: 1200px) but is centered..container-fluid
: Stretches to 100% width..container-narrow
: Ideal for focused content (max-width: 800px)..container-wide
: For expansive layouts (max-width: 1400px)..container-small
: For compact sections (max-width: 600px).
Use Case:
Use .container
when you want your content to be centered with a max-width; choose .container-fluid
for full-width sections (e.g., headers or footers).
3. Rows & Columns
Rows
.row {
display: flex;
flex-wrap: wrap;
gap: var(--grid-gap);
}
Purpose:
The .row
class creates a flex container that wraps its children and spaces them using a consistent gap (set via --grid-gap
). Itâs the building block for horizontal layouts.
Use Case:
Wrap your columns in a .row
to create a horizontal group that automatically wraps on smaller screens.
Grid Columns
[class^="col-"] {
flex: 1 1 0;
min-width: 0;
padding: var(--base-padding);
}
Purpose:
All column classes (like .col-1
through .col-12
) get a base padding and a flexible base. The columns are calculated based on a 12âcolumn layout.
Specific Column Widths
.col-1 { flex: 0 0 calc(100% / var(--grid-columns) * 1); max-width: calc(100% / var(--grid-columns) * 1); }
...
.col-12 { flex: 0 0 calc(100% / var(--grid-columns) * 12); max-width: calc(100% / var(--grid-columns) * 12); }
Purpose:
These classes allow you to set an elementâs width as a fraction of the total container width. For example, .col-6
takes up 50% of the width.
Use Case:
Use these when you need fixed-width columns. For instance, if you want a sidebar and content area, you might use .col-3
for the sidebar and .col-9
for the content.
Auto Layout Column
.col-auto {
flex: 1 1 auto;
max-width: 100%;
}
Purpose:
Allows an element to grow and shrink as needed, taking up available space.
Use Case:
Use .col-auto
for items that should fill any remaining space in a row.
4. Spacing Utilities
Margin Utilities
.m-0 { margin: 0; }
.m-1 { margin: 5px; }
.m-2 { margin: 10px; }
.m-3 { margin: 15px; }
.m-4 { margin: 20px; }
.m-5 { margin: 30px; }
Purpose:
These utility classes allow you to quickly adjust the margin on an element.
Vertical & Horizontal Margins
.mv-0 { margin-top: 0; margin-bottom: 0; }
.mv-1 { margin-top: 5px; margin-bottom: 5px; }
...
.mh-0 { margin-left: 0; margin-right: 0; }
.mh-1 { margin-left: 5px; margin-right: 5px; }
...
Purpose:
Separate utilities for vertical (mv-*
) and horizontal (mh-*
) margins provide more granular control.
Padding Utilities
.p-0 { padding: 0; }
.p-1 { padding: 5px; }
.p-2 { padding: 10px; }
.p-3 { padding: 15px; }
.p-4 { padding: 20px; }
.p-5 { padding: 30px; }
Purpose:
These classes let you add consistent padding to elements.
Directional Padding
.pv-0 { padding-top: 0; padding-bottom: 0; }
.pv-1 { padding-top: 5px; padding-bottom: 5px; }
...
.ph-0 { padding-left: 0; padding-right: 0; }
.ph-1 { padding-left: 5px; padding-right: 5px; }
...
Purpose:
Separate utilities for vertical (pv-*
) and horizontal (ph-*
) padding provide finer control.
Use Case (Spacing):
When you need to adjust spacing without modifying element styles directly, use these utility classes. For instance, add .mb-2
to a heading to give it extra bottom margin.
5. Responsive Grid (Mobile-First)
Media Queries for Responsive Columns
Responsive classes ensure that your grid adapts to different screen sizes.
Small Screens (sm):
@media (max-width: 576px) {
.col-sm-12 { flex: 0 0 100%; max-width: 100%; }
.col-sm-6 { flex: 0 0 50%; max-width: 50%; }
.col-sm-4 { flex: 0 0 33.3333%; max-width: 33.3333%; }
.col-sm-3 { flex: 0 0 25%; max-width: 25%; }
}
Medium Screens (md):
@media (max-width: 768px) {
.col-md-12 { flex: 0 0 100%; max-width: 100%; }
.col-md-6 { flex: 0 0 50%; max-width: 50%; }
.col-md-4 { flex: 0 0 33.3333%; max-width: 33.3333%; }
.col-md-3 { flex: 0 0 25%; max-width: 25%; }
}
Large Screens (lg):
@media (max-width: 992px) {
.col-lg-12 { flex: 0 0 100%; max-width: 100%; }
.col-lg-6 { flex: 0 0 50%; max-width: 50%; }
.col-lg-4 { flex: 0 0 33.3333%; max-width: 33.3333%; }
.col-lg-3 { flex: 0 0 25%; max-width: 25%; }
}
Extra Large Screens (xl):
@media (min-width: 1200px) {
.col-xl-12 { flex: 0 0 100%; max-width: 100%; }
.col-xl-6 { flex: 0 0 50%; max-width: 50%; }
.col-xl-4 { flex: 0 0 33.3333%; max-width: 33.3333%; }
.col-xl-3 { flex: 0 0 25%; max-width: 25%; }
}
Purpose:
These media queries allow you to set different column behaviors at various breakpoints. Note that you only see a subset (12, 6, 4, 3) defined here because in many designs those values are the most commonly used for responsive layouts (i.e., full width, half width, one-third width, and one-quarter width).
Use Case:
When designing for mobile devices, you often stack elements vertically (100% width). On tablets and small desktops, you might use two- or three-column layouts. The responsive classes let you adjust the layout easily.
6. Responsive Navigation & Other Layout Fixes
Navigation Fixes
@media (max-width: 768px) {
#menu { display: none; }
#mobile-nav { display: block; float: right; }
}
@media (max-width: 576px) {
#mobile-nav { text-align: center; }
}
Purpose:
Hide the standard menu on smaller screens and show a mobile navigation toggle. This ensures that your navigation is accessible on mobile devices without taking up too much space.
7. Retina Display Fix
Retina Adjustments
@media only screen and (-webkit-min-device-pixel-ratio: 2),
only screen and (min-resolution: 2dppx) {
:root {
--logo-image: url(../images/logo@2x.png);
}
header #logo a {
background-image: var(--logo-image);
background-size: contain;
}
}
Purpose:
Use higher resolution images on devices with high pixel density to ensure sharp visuals.
Use Case:
Retina displays such as modern smartphones and high-end laptops will load the 2x image for a crisper logo.
Use Cases & Best Practices
When to Use Containers
- Multiple Containers:
Use separate container classes to group different sections of your layout. For example, you might have a.container
for your main content, a.container-fluid
for your header or footer, and a.container-narrow
for sidebars or featured content. - Single Container vs. Multiple Containers:
Generally, a single container is used per section of content. Using multiple containers on one page is acceptable if they represent logically distinct content areas.
When to Use Rows and Columns
- Rows:
Wrap columns in a.row
to ensure proper horizontal alignment and spacing. - Columns:
Use specific column classes (e.g.,.col-6
or.col-md-4
) to control the width of elements within a row. This helps in designing responsive layouts where different sections may need to have different widths. - Auto Layout Columns:
Use.col-auto
when you want the element to automatically adjust its width based on its content while still participating in the flex layout.
Spacing Utilities
- Margins and Padding:
Use utility classes (.m-1
,.p-2
, etc.) to quickly adjust spacing without writing custom CSS for each element. This keeps your styles DRY (Donât Repeat Yourself) and speeds up development. - Directional Spacing:
Use.mh-*
and.mv-*
to control horizontal and vertical margins, respectively. Similarly,.ph-*
and.pv-*
control padding.
Layout & Flow Utilities
- Flex Utilities:
Classes such as.flex-row
,.flex-center
, and.order-first
allow for flexible layout adjustments. Theyâre particularly useful for complex components where you might need to change the order or alignment of elements dynamically. - Grid Utilities:
The grid classes (e.g.,.grid-cols-3
,.grid-rows-2
) help you define layouts that arenât strictly linear. Use these when building multi-dimensional layouts.
Responsive Order & Visibility
- Responsive Ordering:
Classes like.order-md-first
or.order-sm-last
let you change the order of items on different screen sizes. This is helpful when you need a different layout for mobile compared to desktop. - Visibility Helpers:
Use classes such as.d-none
or.d-flex
to control element visibility. This is useful for showing or hiding navigation elements, sidebars, or extra content based on screen size.
Example Usage
HTML Example
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Fluid-First Grid System Demo</title>
<link rel="stylesheet" href="grid.css">
<link rel="stylesheet" href="style.css">
</head>
<body>
<!-- Main Container -->
<div class="container">
<!-- Header Row -->
<div class="row align-center">
<div class="col-12">
<header>
<h1 class="text-center">My Website</h1>
</header>
</div>
</div>
<!-- Navigation Row -->
<div class="row">
<nav class="col-12 flex-center">
<a href="#" class="m-1 p-1">Home</a>
<a href="#" class="m-1 p-1">About</a>
<a href="#" class="m-1 p-1">Services</a>
<a href="#" class="m-1 p-1">Contact</a>
</nav>
</div>
<!-- Content Row -->
<div class="row">
<div class="col-3">
<aside class="p-3">
<h2>Sidebar</h2>
<p>Additional links or content go here.</p>
</aside>
</div>
<div class="col-9">
<section class="p-3">
<h2>Main Content</h2>
<p>This is the primary content area, which uses 9 of the 12 columns.</p>
</section>
</div>
</div>
</div>
<!-- Responsive Example for a Portfolio Filter -->
<div class="container">
<div class="row">
<div class="col-12">
<nav class="work-nav flex-center">
<ul class="project-filter">
<li class="type-work">Type of Work</li>
<li><a href="#" data-filter="*" class="selected">All Projects</a></li>
<li><a href="#" data-filter=".wordpress">Wordpress</a></li>
<li><a href="#" data-filter=".snippets">Snippets</a></li>
<li><a href="#" data-filter=".video">Video</a></li>
</ul>
</nav>
</div>
</div>
</div>
</body>
</html>
When to Use Each Utility:
- Container Classes:
Use a.container
to wrap major sections of your page (e.g., header, content, footer). For a full-width section, use.container-fluid
. - Row & Column Classes:
Place.row
around groups of columns. Then use specific classes (e.g.,.col-3
,.col-9
) to create your layout. - Spacing Utilities:
Apply margin and padding classes (e.g.,.m-3
,.p-2
) to elements to create space between them without writing custom CSS. - Flex & Grid Utilities:
When you need to change the direction of elements (e.g., for a navigation menu on mobile), use.flex-column
or.flex-row-reverse
. Use grid utilities when you want to create a grid layout thatâs not strictly flex-based. - Responsive Order & Visibility:
Adjust the order of elements on different screen sizes with classes like.order-md-first
or.order-sm-last
. Hide or display elements with.d-none
,.d-block
, etc.
This grid system is designed to be fluid, modular, and highly responsive. It provides you with a robust set of utilities that can be mixed and matched to build complex layouts while maintaining a DRY (Don’t Repeat Yourself) codebase. By leveraging CSS variables, flexible container designs, and responsive utilities, you can easily adapt your layout to different devices and screen sizes.
Feel free to customize the variables and utility classes further to suit your projectâs unique needs. This documentation should serve as both a reference and a guide for building and maintaining consistent, responsive layouts across your site.
Happy coding!
/* ================================= */
/* --- FLUID-FIRST GRID SYSTEM --- */
/* ================================= */
/* --- Global Reset (Best Practice) --- */
html {
box-sizing: border-box;
}
*, *::before, *::after {
box-sizing: inherit;
margin: 0;
padding: 0;
}
/* ================================== */
/* --- Global Variables --- */
/* ================================== */
:root {
--container-width: 100%;
--container-max-width: 1200px; /* Default max width */
--container-fluid-max-width: 100%;
--grid-gap: 20px; /* Default gap between columns */
--grid-columns: 12; /* Defines a 12-column layout */
--base-padding: 1rem;
}
/* ================================== */
/* --- Container System --- */
/* ================================== */
/* --- Base Container Styles --- */
.container,
.container-fluid,
.container-narrow,
.container-wide {
width: var(--container-width);
margin: 0 auto;
padding-left: var(--grid-gap);
padding-right: var(--grid-gap);
}
/* --- Default Container --- */
.container {
max-width: var(--container-max-width);
}
/* --- Fluid Container (Full Width) --- */
.container-fluid {
max-width: var(--container-fluid-max-width);
}
/* --- Narrow Container (For focused content) --- */
.container-narrow {
max-width: 800px;
}
/* --- Wide Container (For large layouts) --- */
.container-wide {
max-width: 1400px;
}
/* --- Small Container (For compact content) --- */
.container-small {
max-width: 600px;
}
/* --- No Padding Containers --- */
.container-no-padding {
padding-left: 0;
padding-right: 0;
}
/* --- Extra Padding Containers --- */
.container-extra-padding {
padding-left: calc(var(--grid-gap) * 2);
padding-right: calc(var(--grid-gap) * 2);
}
/* --- Responsive Containers --- */
@media (max-width: 768px) {
.container,
.container-fluid,
.container-narrow,
.container-wide,
.container-small {
padding-left: calc(var(--grid-gap) / 2);
padding-right: calc(var(--grid-gap) / 2);
}
}
/* ================================== */
/* --- Grid System --- */
/* ================================== */
/* --- Rows --- */
.row {
display: flex;
flex-wrap: wrap;
gap: var(--grid-gap);
}
/* --- Grid Columns --- */
[class^="col-"] {
flex: 1 1 0;
min-width: 0;
padding: var(--base-padding);
}
/* --- Specific Column Widths (1 to 12) --- */
.col-1 { flex: 0 0 calc(100% / var(--grid-columns) * 1); max-width: calc(100% / var(--grid-columns) * 1); }
.col-2 { flex: 0 0 calc(100% / var(--grid-columns) * 2); max-width: calc(100% / var(--grid-columns) * 2); }
.col-3 { flex: 0 0 calc(100% / var(--grid-columns) * 3); max-width: calc(100% / var(--grid-columns) * 3); }
.col-4 { flex: 0 0 calc(100% / var(--grid-columns) * 4); max-width: calc(100% / var(--grid-columns) * 4); }
.col-5 { flex: 0 0 calc(100% / var(--grid-columns) * 5); max-width: calc(100% / var(--grid-columns) * 5); }
.col-6 { flex: 0 0 calc(100% / var(--grid-columns) * 6); max-width: calc(100% / var(--grid-columns) * 6); }
.col-7 { flex: 0 0 calc(100% / var(--grid-columns) * 7); max-width: calc(100% / var(--grid-columns) * 7); }
.col-8 { flex: 0 0 calc(100% / var(--grid-columns) * 8); max-width: calc(100% / var(--grid-columns) * 8); }
.col-9 { flex: 0 0 calc(100% / var(--grid-columns) * 9); max-width: calc(100% / var(--grid-columns) * 9); }
.col-10 { flex: 0 0 calc(100% / var(--grid-columns) * 10); max-width: calc(100% / var(--grid-columns) * 10); }
.col-11 { flex: 0 0 calc(100% / var(--grid-columns) * 11); max-width: calc(100% / var(--grid-columns) * 11); }
.col-12 { flex: 0 0 calc(100% / var(--grid-columns) * 12); max-width: calc(100% / var(--grid-columns) * 12); }
/* --- Auto Layout Column --- */
.col-auto {
flex: 1 1 auto;
max-width: 100%;
}
/* ================================== */
/* --- Spacing Utilities --- */
/* ================================== */
/* --- Margin Utilities --- */
.m-0 { margin: 0; }
.m-1 { margin: 5px; }
.m-2 { margin: 10px; }
.m-3 { margin: 15px; }
.m-4 { margin: 20px; }
.m-5 { margin: 30px; }
/* Vertical Margin (Top & Bottom) */
.mv-0 { margin-top: 0; margin-bottom: 0; }
.mv-1 { margin-top: 5px; margin-bottom: 5px; }
.mv-2 { margin-top: 10px; margin-bottom: 10px; }
.mv-3 { margin-top: 15px; margin-bottom: 15px; }
.mv-4 { margin-top: 20px; margin-bottom: 20px; }
.mv-5 { margin-top: 30px; margin-bottom: 30px; }
/* Horizontal Margin (Left & Right) */
.mh-0 { margin-left: 0; margin-right: 0; }
.mh-1 { margin-left: 5px; margin-right: 5px; }
.mh-2 { margin-left: 10px; margin-right: 10px; }
.mh-3 { margin-left: 15px; margin-right: 15px; }
.mh-4 { margin-left: 20px; margin-right: 20px; }
.mh-5 { margin-left: 30px; margin-right: 30px; }
/* --- Padding Utilities --- */
.p-0 { padding: 0; }
.p-1 { padding: 5px; }
.p-2 { padding: 10px; }
.p-3 { padding: 15px; }
.p-4 { padding: 20px; }
.p-5 { padding: 30px; }
/* Vertical Padding */
.pv-0 { padding-top: 0; padding-bottom: 0; }
.pv-1 { padding-top: 5px; padding-bottom: 5px; }
.pv-2 { padding-top: 10px; padding-bottom: 10px; }
.pv-3 { padding-top: 15px; padding-bottom: 15px; }
.pv-4 { padding-top: 20px; padding-bottom: 20px; }
.pv-5 { padding-top: 30px; padding-bottom: 30px; }
/* Horizontal Padding */
.ph-0 { padding-left: 0; padding-right: 0; }
.ph-1 { padding-left: 5px; padding-right: 5px; }
.ph-2 { padding-left: 10px; padding-right: 10px; }
.ph-3 { padding-left: 15px; padding-right: 15px; }
.ph-4 { padding-left: 20px; padding-right: 20px; }
.ph-5 { padding-left: 30px; padding-right: 30px; }